What is InteractionLib?
InteractionLib, as with most of my side-projects, was formed out of necessity. My team at work is responsible for pumping out small web-based corporate tooling for internal functions. In my line of work it is necessary to constantly find ways to optimize process and increase productivity.
Today web-based applications are common and tooling for productivity is plentiful. So the question is: Why am I not using an all-inclusive framework package? Solid names like Telerik may immediately come to mind - and don't get me wrong, those guys over there are pretty damn smart - but their products, like so many others, are their product with their look and feel. So the answer to the question is: I work for Blizzard.
And just as with Blizzard external products, Blizzard internal products require a certain level of awesome. I am not in any way saying that these aforementioned products don't emanate all sorts of awesome, but they are a very specific awesome that commonly require more time and work to customize to a Blizzard designer's look and feel.

InteractionLib attempts to accomplish this by not only streamlining the wire up of client-side interactions, but also making back-and-forth server-client communications literally take seconds to implement. With InteractionLib I am able to wire up complex single-page-applications (SPA) with almost no effort and in even less time.
By creating a framework around current best patterns & practices InteractionLib provides a clean and maintainable method for me to eliminate time-consuming configurations and focus on meaningful, presentable code.
Let's take a look at one common configuration of apps-from-scratch out in the wild:
What this typically translates to is a page which has its own "configuration" .js file. That file will find various IDs and classes on the page and wire these up the to appropriate plugins and create the appropriate interactions for the page.
Individually these little interaction wire-ups are small and inconsequential. But a single page will have a number of these interactions, each adding up to a significant amount of time and repetitive code. I've actually worked with developer's in the past who would literally write the same block of javascript over and over again without a second thought to efficiency and maintainability.
What InteractionLib does for me is completely cuts out the middle man. In many cases InteractionLib can handle the interaction all on its own leaving even less code to be written.
Additionally InteractionLib allows my pages to pick and choose what they need to be wired up to. More importantly is it also allows me to do my wire ups directly in my markup making my page more descriptive and ultimately more maintainable.
To demonstrate this take a look at the wire-up below for a custom plugin:
- <div class="my-plugin plugin-option-1 plugin-option-2"></div>
- $('.my-plugin').each(function () {
- var element = $(this);
- var option1 = element.hasClass('plugin-option-1');
- var option2 = element.hasClass('plugin-option-2');
- element.myPlugin({ option1: option1, option2: option2 });
- });
Below is the InteractionLib way to wire this plugin up:
- <div data-interaction="myPlugin"
- data-configuration-option1="true"
- data-configuration-option2="true"></div>
- I no longer need to write any wire-up code, nor do I have to need an extra .js file to store it in
- I can come back to this project years later and immediately recognize exactly what is wired up here and how
- I no longer have to worry about a designer attaching styles to a class on my element that was meant for functionality
- My wire-ups here also respond to ajaxed content that comes in after the page has finished loading (SPAs).
Now, I also said that InteractionLib handles server communications. It does this by wrapping the jQuery AJAX library and exposing it through a number of easy-to-use data attributes. Before I get into these attributes, though, I want to discuss the basic workflow that InteractionLib is trying to encapsulate.
The process of a user interaction can be broken down into these four simple parts. The user does something, the page sends a query to the server, the server returns a response, and the page inserts the response.
Effectively what this breaks down to in the web is a "form" that uses AJAX instead of an actual form post, and then has a designated space or way to display the resulting HTML or JSON returned from the server.
A very common example of this would be a search text input that sends the search results back to the server. The server then sends back a block of HTML rows (<tr>) containing the search results. The resulting HTML then replaces the content that was previously in the body of the table displaying the results. Each time the user changes the search text, the results are updated without making a complete page-update and only loading the minimal amount of HTML to display results.
The old way of doing this required an ID or some identifier that could easily be mistaken by a designer as something that can be renamed, removed, or used for styling. That ID would be searched for by some javascript hidden in a .js file somewhere that is difficult to find year(s) later. Once found, a keydown event would be attached to the text input that would wait until the enter key was pressed. When an enter key has been detected, the text from the input is sent to a hard-coded address on the server which will likely change one day leaving this functionality magically broken. After a response is returned from the server, the javascript will find the place on the page where the results must go, clear out any previous results, and place the new results there.
The little chunk of code for doing all this would be around 10 - 40 lines of jQuery AJAX vomit, repeated half a dozen times per page for every user interaction like this. Of course the developer comments and optimizes the code the best they can, but ultimately time will be spent the next time this application is updated reading through the sewer that is this application's client-side code.
This is where InteractionLib will make the biggest impact.
- <!-- using just ajaxform-action and ajaxform-target -->
- <!-- this entire interaction is taken care of -->
- <!-- using no additional javascript -->
- <form data-ajaxform-action="Some/Server/Action"
- data-ajaxform-target="#server-response">
- <label>
- Search Criteria
- <input type="text" name="criteria"/>
- </label>
- <button type="submit">Search</button>
- </form>
- <table>
- <tbody id="server-response">
- <!-- The resulting rows from the server -->
- <!-- will be placed here (ajaxform-target) -->
- </tbody>
- </table>
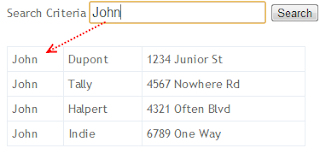
If you would like to know more about InteractionLib you can find the project on GitHub.com, Nuget.org, and the documentation at markonthenet.com/InteractionLib.
You can also contact me on markonthenet.com/Me if you have any questions, comments, or requests for InteractionLib.